ESP32 with Soil Moisture Sensor and LCD using MicroPython
Monitor Soil Moisture with ESP32 board and Soil Moisture Sensor Module with MicroPython. Show Status on LCD Display.
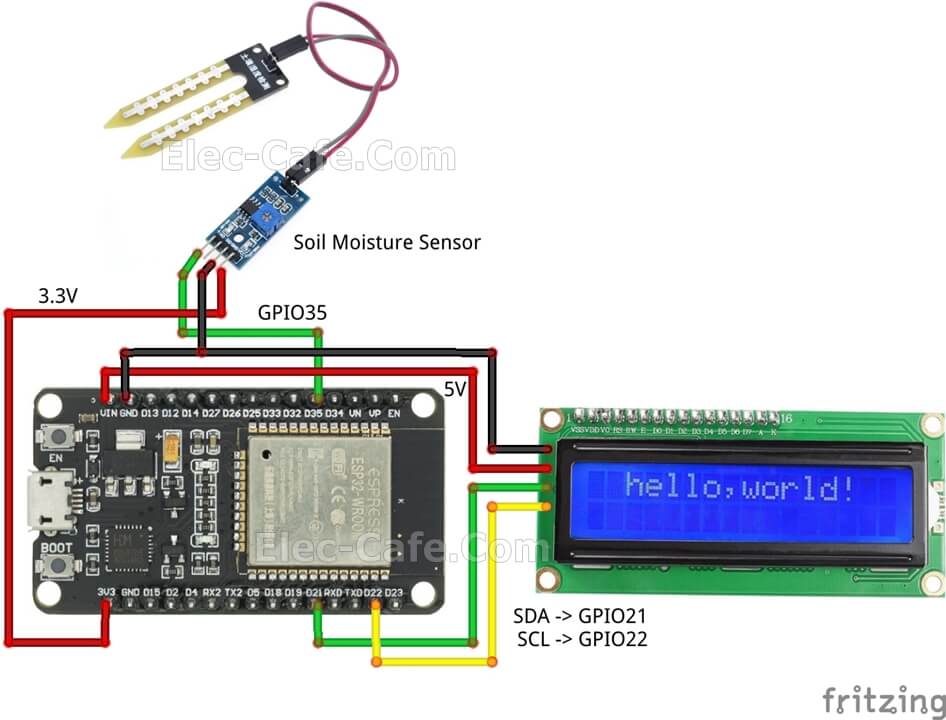
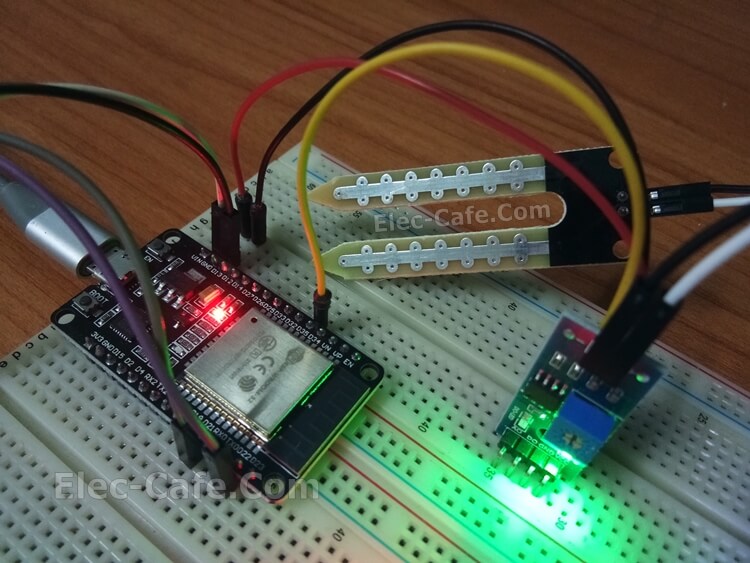
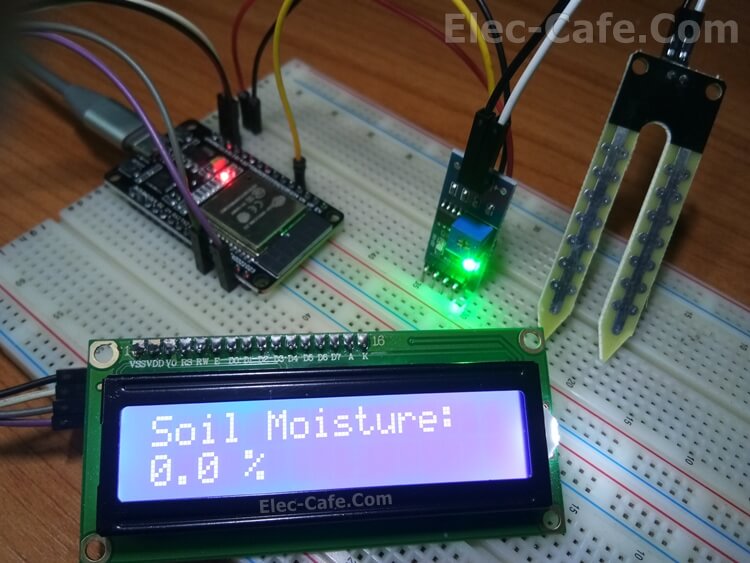
MicroPython Code – ESP32 with Soil Moisture Sensor and LCD Display
from machine import Pin, SoftI2C
from machine import Pin, ADC
from i2c_lcd import I2cLcd
import time
# LCD
i2c = SoftI2C(scl=Pin(22), sda=Pin(21),freq=100000)
lcd = I2cLcd(i2c, 0x27,2,16)
time.sleep(1)
lcd.clear()
# Soil Moisture
soil = ADC(Pin(35))
m = 100
min_moisture=0
max_moisture=4095
soil.atten(ADC.ATTN_11DB) #Full range: 3.3v
soil.width(ADC.WIDTH_12BIT) #range 0 to 4095
# START
text = 'Starting...'
lcd.putstr(text)
while True:
try:
soil.read()
time.sleep(2)
m = (max_moisture-soil.read())*100/(max_moisture-min_moisture)
moisture = '{:.1f} %'.format(m)
print('Soil Moisture:', moisture)
lcd.clear()
lcd.putstr('Soil Moisture: ')
lcd.move_to(0,1)
lcd.putstr(moisture)
time.sleep(5)
except:
pass