ESP32 Auto Control Pump using Soil Moisture Sensor With LCD (MicroPython)
Control Water Pump Automatic with MicroPython. Using ESP32 board and Soil Moisture Sensor. Turn Water Pump on if Soil Moisture Low Level. Turn Water Pump off if Soil Moisture High Level. Show status on LCD Display.
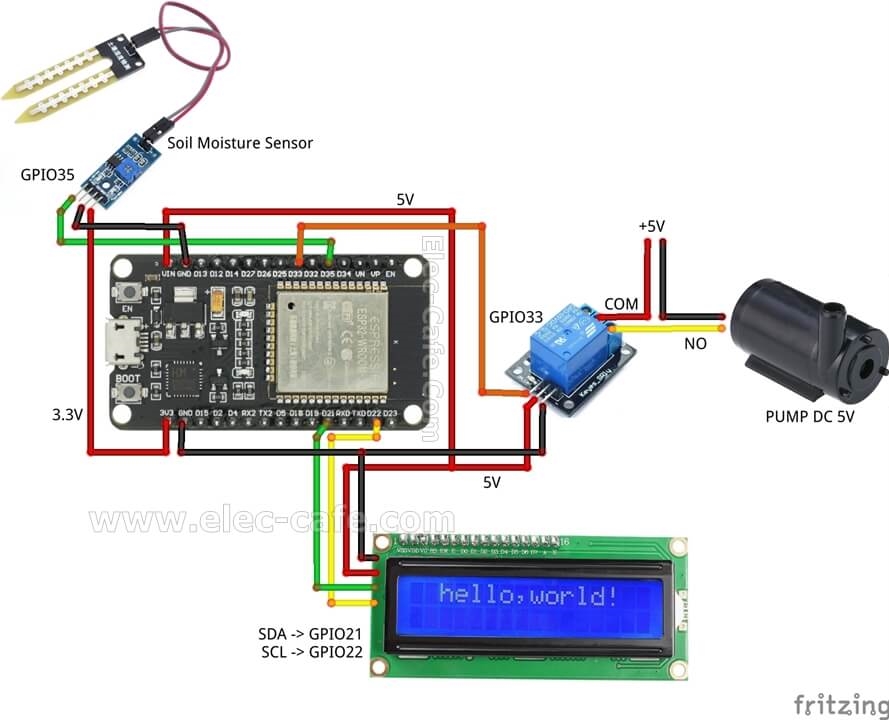
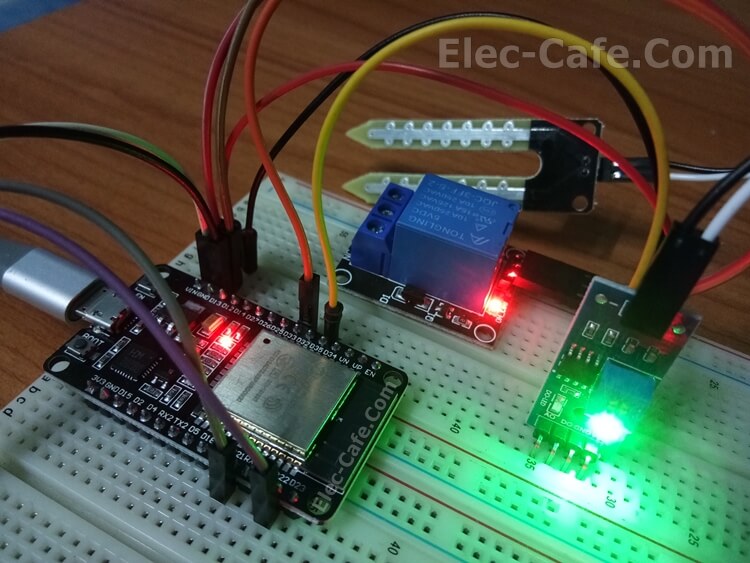
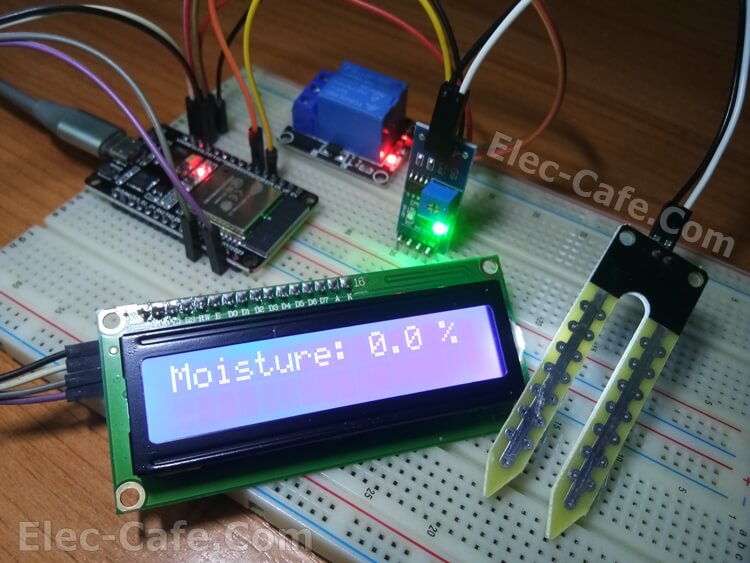
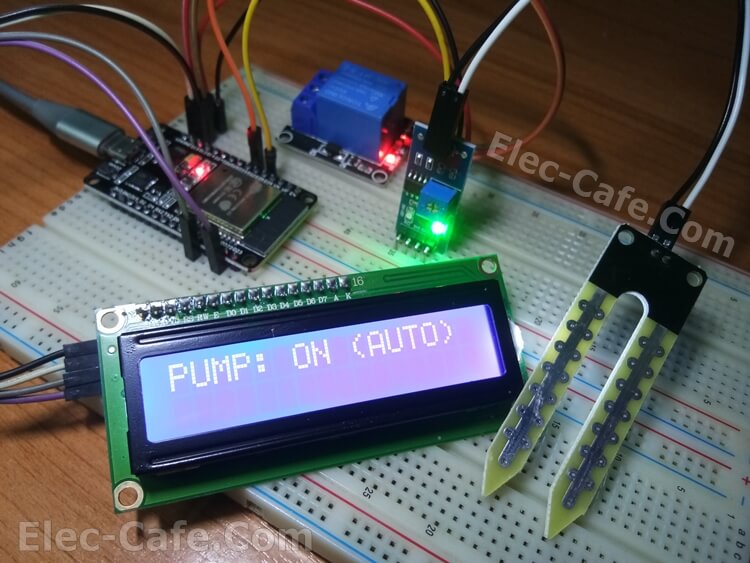
MicroPython Code – ESP32 Auto Control Pump using Soil Moisture Sensor With LCD Display
from machine import Pin, SoftI2C
from machine import Pin, ADC
from i2c_lcd import I2cLcd
import time
import _thread
# PUMP
pump = Pin(33, Pin.OUT)
pump.off()
# LCD
i2c = SoftI2C(scl=Pin(22), sda=Pin(21),freq=100000)
lcd = I2cLcd(i2c, 0x27,2,16)
time.sleep(1)
lcd.clear()
# Soil Moisture
soil = ADC(Pin(35))
m = 100
min_moisture=0
max_moisture=4095
soil.atten(ADC.ATTN_11DB) #Full range: 3.3v
soil.width(ADC.WIDTH_12BIT) #range 0 to 4095
def check_moisture():
print('Check Moisture Starting...')
global m
while True:
try:
soil.read()
time.sleep(2)
m = (max_moisture-soil.read())*100/(max_moisture-min_moisture)
moisture = 'Soil Moisture: {:.1f} %'.format(m)
print("Soil Moisture: " + "%.1f" % m +"% (adc: "+str(soil.read())+")")
time.sleep(5)
except:
pass
# START
text = 'Starting...'
lcd.putstr(text)
# RUN
global pump_status
pump_status = 'OFF'
def show_lcd():
while True:
try:
moisture = 'Moisture: {:.1f} %'.format(m)
pump_s = 'PUMP: {:}'.format(pump_status)
lcd.clear()
lcd.putstr(pump_s)
time.sleep(2)
lcd.clear()
lcd.putstr(moisture)
time.sleep(2)
except:
pass
def auto_pump():
global pump_status
while True:
if m < 20:
time.sleep(1)
pump.on()
pump_status = 'ON (AUTO)'
time.sleep(5) # TURN ON PUMP 5 sec
pump.off()
pump_status = 'OFF (AUTO)'
time.sleep(10)
else:
pass
_thread.start_new_thread(check_moisture,())
_thread.start_new_thread(show_lcd,())
_thread.start_new_thread(auto_pump,())